I have used OData operators like $select, $filter and $expand in previous posts (REST in SharePoint) without any real explanation. This post is the first in a series that will rectify that by giving detailed descriptions of how to use OData operators in SharePoint REST requests. In this post, I’m going to explain the purpose and usage of two OData operators, $select and $expand.
The Base Query
Throughout this post, I’m going to be querying a picture library called ‘Pictures’. Rather than repeat the same query a dozen times, I’m going to go over the basic query here and then show how I can modify it by supplying OData operators as query parameters. I’m going to be running these queries in the browser console, and my query will use $.ajax, so I need to make sure jQuery is loaded into the page. To do that, I’ll run the following code, which inserts a script tag into the page that loads jQuery from a CDN:
1 2 3 4 | var script = document.createElement('script'); script.type = 'text/javascript'; script.src = 'https://cdn.jsdelivr.net/npm/jquery@1.12.4/dist/jquery.js'; document.head.appendChild(script); |
And here is the base query, which retrieves all items from the picture list:
1 2 3 4 5 6 7 8 9 10 11 12 | var url = _spPageContextInfo.webAbsoluteUrl + "/_api/Web/Lists/getByTitle('Pictures')/Items"; $.ajax({ url: url, async: false, headers: { 'accept': 'application/json;odata=nometadata' }, complete : function(json) { console.log(json.responseJSON); } }); |
And the result returned from this query looks like so:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | { "value": [ { "FileSystemObjectType": 0, "Id": 333, "ServerRedirectedEmbedUri": null, "ServerRedirectedEmbedUrl": "", "ContentTypeId": "0x0101020013D6336EAB2A0B4981C0EE30DB34E49F", "Title": "Poipu - Hawaii", "ImageWidth": 800, "ImageHeight": 550, "ImageCreateDate": "2010-11-03T14:01:32Z", "Description": "Snorkeling", "Keywords": null, "ComplianceAssetId": null, "MediaServiceAutoTags": null, "ID": 333, "Created": "2017-04-07T14:39:31Z", "AuthorId": 10, "Modified": "2018-01-11T21:22:48Z", "EditorId": 10, "OData__CopySource": null, "CheckoutUserId": null, "OData__UIVersionString": "1.0", "GUID": "6c893f4f-b490-48b9-bf7d-494f826bb6ff" }, {...}, {...} ] } |
It’s just an object with a property called value, which is an array of objects. Each object in the array represents a single item, with a property for each field of the item. This result is not ideal. There’s a lot of crap in there that I probably don’t care about. Like what the heck is a ‘MediaServiceAutoTags’ or a ‘ComplianceAssetId’? In the next section I’ll show how to use $select to limit the fields that are returned to only what I care about. This will reduce the load on the server, the network traffic, and even the load on the browser.
Using $select to Narrow Results
$select is an OData operator. You specify OData operators by tacking a query string onto the end of the request URL, which is just a question mark, followed by one or more name equals value pairs separated by ampersands. The value for the $select parameter is one or more field names separated by a comma. The field names must be the internal name of the field, not the display name. So to limit the results of the base query to just the Title and Description fields, I can tack on the following query parameters:
1 | ?$select=Title,Description |
And the results of this query are:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | { "value": [ { "Title": "Poipu - Hawaii", "Description": "Snorkeling" }, { "Title": "Sedona Arizona - Pink Jeep Tour", "Description": "Exploring Woo Hoo!" }, { "Title": "IronHorse Winery - Sonoma", "Description": "Tasting Part Deux" } ] } |
Isn’t that much better? Now I know that many of you have already stopped reading and will forever more run REST queries in SharePoint bringing back all fields. How do I know this? Because of the 25+ years experience fixing bad SQL code with queries like ‘SELECT * FROM WhatEvs’. It’s a bad idea in SQL, and it’s an even worse idea in SharePoint.
Why worse in SharePoint? Because of the ease with which a non-technical user can change the underlying ‘table’ structure. In other words consider this:
- I write some code on the home page of a site that pulls back all items and fields from a list someplace else and displays them. When I write this code, there were 3 items and 2 fields and everything works swimmingly.
- Everybody in my organization goes to this home page first thing in the morning (you can probably already see it coming).
- Long after I’ve moved on to some other job, some SCA adds 20 fields to the list. Some of them are Notes fields (which can contain up to 65kB each). Also, over time, the list as grown to thousands of items.
- Now the home page takes forever to load, and everybody loading it at once brings the server to its knees. Asked why, the SCA says it’s because my code sucks.
And she’s correct; it is because my code sucks. It’s now bringing back tons of crap that it doesn’t even care about, and it’s throwing it away as soon as it gets it. But along the way, it put a considerable load on the server, the network, and the browser. This is a potential issue even in the SQL scenario, but generally only a technical person could have added all that crap to a table, and if they did that without any thought to how that might affect performance then it’s on them at least as much as it’s on me. But in the SharePoint scenario, you really can’t blame the SCA, they’re just using SharePoint the way it was intended to be used. So in general, limit the width of your results by only selecting fields that you’re going to use or display.
The general syntax for $select looks like the following image. Don’t forget the question mark at the end of the base URL, the dollar sign at the beginning of each OData operator, or to put an ampersand separator between each query parameter or you’ll be scratching your head for a while. So select is pretty simple, but it gets a bit more complicated if you need to pull back data from nested objects, which I’ll get into in the next section.
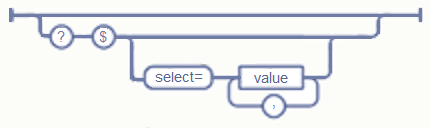
Using $expand with $select to Return Nested/Deferred Complex Properties
What the heck is a nested/deferred complex property? In general, it’s a lookup of some sort. All items contain lookups. For starters, the Author and Editor of the item are both lookups. In the case of my picture library, the items also have a File property, which is really just a lookup to the Web.Files collection of the current site. And of course, you can add fields that are lookups to other lists.
And why are lookups deferred? You may know that there is a practical limit to how many lookups you can display in a given view in SharePoint before you can expect to run into performance problems. That’s not a front-end limit. When you expand lookups, you’re effectively doing a SQL JOIN. Too many JOINS can lead to huge result sets and bog down SQL server leading to slow results and possibly farm-wide performance problems.
To provide the flexibility to avoid these type of bottlenecks, the back-end doesn’t expand lookups unless you ask it too, which is the purpose of the $expand operator. The following query string will expand the File property:
1 | ?$expand=File |
And the result of this looks like:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | { "value": [ { "File": { "CheckInComment": "", "CheckOutType": 2, "ContentTag": "{F1DDE157-D0CC-45F8-B2D5-7E8D78AEB32A},4,7", "CustomizedPageStatus": 0, "ETag": "\"{F1DDE157-D0CC-45F8-B2D5-7E8D78AEB32A},4\"", "Exists": true, "IrmEnabled": false, "Length": "476492", "Level": 1, "LinkingUri": null, "LinkingUrl": "", "MajorVersion": 1, "MinorVersion": 0, "Name": "Poipu_Hawaii.jpg", "ServerRelativeUrl": "/sites/csrdemos/Pictures/Poipu_Hawaii.jpg", "TimeCreated": "2017-04-07T14:39:31Z", "TimeLastModified": "2018-01-11T21:22:49Z", "Title": "Poipu - Hawaii", "UIVersion": 512, "UIVersionLabel": "1.0", "UniqueId": "f1dde157-d0cc-45f8-b2d5-7e8d78aeb32a" }, "FileSystemObjectType": 0, "Id": 333, "ServerRedirectedEmbedUri": null, "ServerRedirectedEmbedUrl": "", "ContentTypeId": "0x0101020013D6336EAB2A0B4981C0EE30DB34E49F", "Title": "Poipu - Hawaii", "ImageWidth": 800, "ImageHeight": 550, "ImageCreateDate": "2010-11-03T14:01:32Z", "Description": "Snorkeling", "Keywords": null, "ComplianceAssetId": null, "MediaServiceAutoTags": null, "ID": 333, "Created": "2017-04-07T14:39:31Z", "AuthorId": 10, "Modified": "2018-01-11T21:22:48Z", "EditorId": 10, "OData__CopySource": null, "CheckoutUserId": null, "OData__UIVersionString": "1.0", "GUID": "6c893f4f-b490-48b9-bf7d-494f826bb6ff" }, {...}, {...} ] } |
Again, I’m not selecting anything, so the service is returning everything. Not just all properties of the items but also all properties of the File. To narrow the results, I need to add a $select, like so:
1 | ?$expand=File&$select=Title,Description |
I’ve added back the same $select value I used in the last section, and now I get back the item Title and Description, but I also get back the File properties with all it’s sub-properties.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | { "value": [ { "File": { "CheckInComment": "", "CheckOutType": 2, "ContentTag": "{F1DDE157-D0CC-45F8-B2D5-7E8D78AEB32A},4,7", "CustomizedPageStatus": 0, "ETag": "\"{F1DDE157-D0CC-45F8-B2D5-7E8D78AEB32A},4\"", "Exists": true, "IrmEnabled": false, "Length": "476492", "Level": 1, "LinkingUri": null, "LinkingUrl": "", "MajorVersion": 1, "MinorVersion": 0, "Name": "Poipu_Hawaii.jpg", "ServerRelativeUrl": "/sites/csrdemos/Pictures/Poipu_Hawaii.jpg", "TimeCreated": "2017-04-07T14:39:31Z", "TimeLastModified": "2018-01-11T21:22:49Z", "Title": "Poipu - Hawaii", "UIVersion": 512, "UIVersionLabel": "1.0", "UniqueId": "f1dde157-d0cc-45f8-b2d5-7e8d78aeb32a" }, "Title": "Poipu - Hawaii", "Description": "Snorkeling" }, {...}, {...} ] } |
To limit the File properties, I need to select something inside the file. For example, to select the File.Name, I can added File/Name to the $select, like so:
1 | ?$expand=File&$select=Title,Description,File/Name |
And the result, shown below, contains just what I need. Much better!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | { "value": [{ "File": { "Name": "Poipu_Hawaii.jpg" }, "Title": "Poipu - Hawaii", "Description": "Snorkeling" }, { "File": { "Name": "PinkJeep_Sedona.jpg" }, "Title": "Sedona Arizona - Pink Jeep Tour", "Description": "Exploring Woo Hoo!" }, { "File": { "Name": "IronHorse_Sonoma.jpg" }, "Title": "IronHorse Winery - Sonoma", "Description": "Tasting Part Deux" } ] } |
You can expand multiple lookups in a single query. Here, I’ve added Author to the $expand operator:
1 | $select=Title,Description,File/Name&$expand=File,Author |
And the result looks like:
1 2 3 4 5 6 7 8 9 10 | { "odata.error": { "code": "-1, Microsoft.SharePoint.SPException", "message": { "lang": "en-US", "value": "The query to field 'Author' is not valid. The $select query string must specify the target fields" + " and the $expand query string must contains Author." } } } |
Um…wait…that doesn’t look right? The problem here is well documented in the Microsoft post Use OData query operations in SharePoint REST requests. You cannot expand something without also selecting something inside of it. Of course, I just did exactly that with the File object at the beginning of this section, and it just returned all properties of the file? That is an undocumented anomaly. In general if you expand something you must select something inside of it or you will get an error similar to the one above. Fixing that by selecting Author/LastName, I get:
1 | $select=Title,Description,File/Name,Author/LastName&$expand=File,Author |
And the result looks like:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | { "value": [ { "File": { "Name": "Poipu_Hawaii.jpg" }, "Author": { "LastName": "McShea" }, "Title": "Poipu - Hawaii", "Description": "Snorkeling" }, { "File": { "Name": "PinkJeep_Sedona.jpg" }, "Author": { "LastName": "McShea" }, "Title": "Sedona Arizona - Pink Jeep Tour", "Description": "Exploring Woo Hoo!" }, { "File": { "Name": "IronHorse_Sonoma.jpg" }, "Author": { "LastName": "McShea" }, "Title": "IronHorse Winery - Sonoma", "Description": "Tasting Part Deux" } ] } |
So the basic syntax for $expand looks like this:
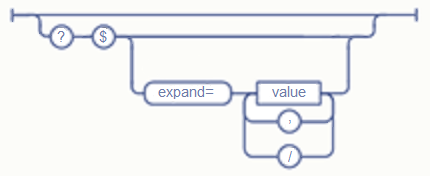
Now here, the value should be an object property. I’ve seen numerous examples where people did things like:
1 | ?$select=Author/LastName,Author/FirstName&$expand=Author/LastName,Author/FirstName |
This syntax actually works, but it is not really correct. You expand objects and select primitives. Author is an object property, and LastName and FirstName are primitive properties, so it really should be:
1 | ?$select=Author/LastName,Author/FirstName&$expand=Author |
The result is actually the same either way, but the first one contains some redundant nonsense. And remember, of course, that if you expand something, you must also select something inside of it.
One quick note about expanding user fields. When you expand a user field, what you are actually expanding is a lookup to the hidden site collection UserInfo list (/_catalogs/users). The fields that are available to select are the fields that are in this list. And the values are brought in from the user’s profile, but can get stale, and not all values in the profile are available from this list. I also read something, available in the references, that said that if a you query for a value that is not populated, you will get an OData error for a response, but that hasn’t been my experience. It may be dependent on the version of SharePoint you are running on.
Summing Up
That’s all I’ve got for $select and $expand for now. I will add to this post as I discover new things since it’s kind of a reference for me as well.
It’s not that complicated, and I mostly muddled along looking at examples and deducing what works for some time before I started looking at the documentation and formally codifying it into a set of rules. In my next post, I’ll take a look at the OData operators $filter and $orderBy.